The API guide
What is an API call?
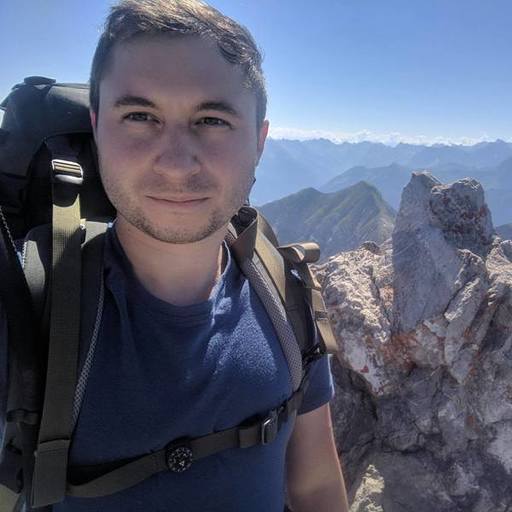
David Fateh
Updated: January 15, 2025
The API guide
This is chapter 4 of the series, The API guide
Summary
An API call is the action that brings APIs to life, enabling applications to request and exchange data seamlessly. Whether you’re fetching information, sending updates, or integrating systems, API calls are the essential mechanism driving communication between services.
In this chapter, we’ll define what an API call is, walk through how to make one, and provide real-world examples to illustrate their role in API architecture. You’ll also learn how to secure API calls, explore their relationship with API infrastructure, and discover ways to simplify and optimize the process.
API calls defined
An API call is a message sent from a client application to a location within another application programming interface in order to trigger a defined action. That action typically entails the transfer of data or functionality to an external program, which could involve:
- Retrieving data on a recent order.
- Generating an invoice or report.
- Sending a pre-written email.
- Executing a purchase or sale.
- Performing a calculation.
- Sending a notification to a messaging platform.
API calls (sometimes referred to as API requests) are integral to the two-way “request-response” communication process that occurs between applications, and so are particularly important in API-first software architectures.
Once the API call is specified, it is sent to an API endpoint within an external server API. If the call is accepted, and valid, the recipient API sends back a response. Most API calls need to include an API key so that the server API can authenticate them, and grant access to the requested data.
As a real-world example, think of an app that displays real-time headlines for a news agency. In order to obtain that real-time information, the app would need to retrieve data from the agency’s own IT infrastructure. To do that, it would formulate a call or request and then send that call to the relevant part of the agency server, via the agency’s API. If the call was successful, the server would send back the relevant response data and then the app could display it as a headline.
It’s helpful to think of the call process like physical mail. An API call to an API endpoint is like a letter sent to a specific address: if the address is correct, and contains all the necessary information, the letter should reach its destination, be read and understood, and then receive a response.
How to make an API call
To build an API call, you’ll need to know the following information:
-
The address — the uniform resource locator (URL) of the API endpoint with optional parameters.
-
The HTTP action — the request type, such as GET or POST.
-
Headers — for adding details and authentication information.
-
Body (also called a payload, optional).
Let’s take a closer look at each component:
-
Address: You need to know the correct URL of the API endpoint in order to send your API call to the right place. You’ll need to write out the call in the form of the URL before you add specifying data (see below) to trigger the relevant action.
-
HTTP Action: You’ll need to add the action that you want your call to trigger to the URL thread. In the context of a REST API, those actions are designated by a set of specific HTTP verbs, including
GET
,POST
,PUT
, andDELETE
. -
Headers: The header includes further detail about both the request and the response that the call is intended to generate. Common header components include “user-agent,” which helps the server identify the API making the call; “content type,” which helps the server understand the content of the call; and “accept,” which denotes the format of response you want to receive. The API key and other authentication information should also be included in the header.
-
Body: Not all requests require a body or payload. However, if you are submitting things like form data, files, or JSON, you will need to provide this data in the body or else the API may respond with an error code.
Every service that processes API calls constantly listens for requests from API clients. The services retrieve client requests, ensure they include the appropriate security data (API keys, headers), unpack the parameters and data used, and then return a response to the client.
API Call Responses
Once you’ve compiled and sent your call, you’ll need to wait for an API response. The API server will typically display a status code to indicate whether the call has been successful or has encountered an error, for example:
-
Success codes are typically indicated by “2xx.” For example, the “200” status code indicates that a call was successful, while “201” indicates that the API has created a resource in response to a POST call.
-
Error codes are typically indicated by “4xx.” For example, a “400” code indicates that the request is invalid, a “401” code indicates that the request is unauthorized, and a “404” code that a requested resource has not been found.
-
Server errors are indicated by “5xx” codes, meaning that the server encountered an unexpected error and developers maintaining that server likely have an infrastructure problem or bug in the server code.
Examples of API calls
Here’s an example of an API call that you can try on your terminal (this functionality is built in to macOS and Linux):
curl https://api.github.com/repos/torvalds/linux
The above example will use the GitHub REST API to return some information about the code of the Linux kernel, which is used on everything from ATMs to space stations. You can make an API call with every programming language.
Let’s look at another example in a more visual way using Postman, a web API platform with a great user interface — and a very useful tool to try out public APIs. Our example will build a request using JSON parameters and headers. Then, Postman will request data and display the response we receive.
To use the Contentful API to retrieve all the Content Types in a given space, we’d use the following endpoint:
https://cdn.contentful.com/spaces/abc12345/content_types
If you enter that endpoint directly as a GET
request in Postman, you’ll receive the following “401 Unauthorized” status and an error message requesting an access token:
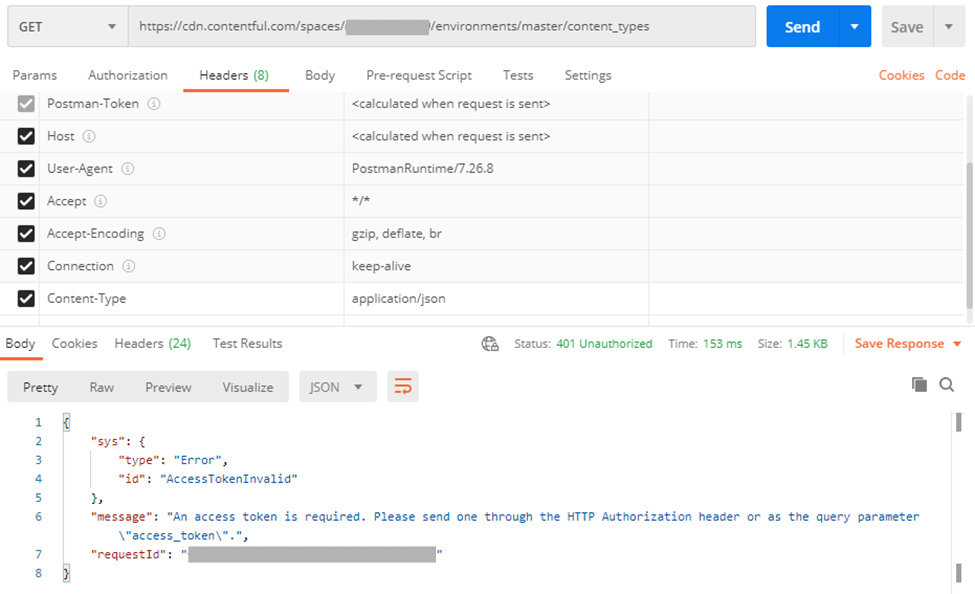
To resolve this error, you’d simply add the access token to the request header — either by using the Authorization tab, or by inputting the token to the header manually.
It’s worth noting that the response header also returns metadata about the endpoint that received the request, including the organization ID, and the region from which the request originated.
Although the example above is from a read-only API, other APIs allow you to push information into the destination system. If you wanted to build a request to create or update data, then you’d take the following actions:
- Change the request type from
GET
toPOST
(or any other HTTP verb). - Add the formatted JSON data to the request body (JSON is one of the most popular data formats).
- Set the request’s Content-Type header to application/json.
Then your data would be sent to the service in the request body.
How do you secure API calls?
Because API calls reach internal locations within server APIs (API endpoints), they can sometimes become a security vulnerability. Attackers may use an API request to compromise a server in a number of ways, including via distributed denial-of-service (DDOS) attacks which flood a target server with requests and prevent other users from accessing its functionality. Alternatively, an attacker may be able to exploit the target API’s code with an invalid API request that triggers a functionality problem.
Fortunately, developers can prevent the malicious use of API keys, and secure their APIs against this kind of incident, by implementing the following strategies:
-
API key: A unique identifier used to authorize and verify API clients, and enable access to an API’s data and functionality.
-
Authentication tokens: API keys can be used in conjunction with authentication tokens in order to strengthen API security against malicious calls.
-
Endpoint tracking: An up-to-date list of all endpoints within an API that helps developers track potential vulnerabilities.
-
DDOS mitigation: Certain strategies (implemented via cloud service or software) can mitigate the effects of a DDOS attack, including blocking incoming API calls.
API calls and API architecture
The examples we’ve used above involve API calls made to a REST API but other types of API architecture may require a different type of call structure. Key examples include:
-
Representational state transfer (REST) API: Calls are made in HTTP and involve inputting URLS with specific verb actions.
-
Simple object access protocol (SOAP) API: Slightly more complex than REST APIs, SOAP APIs require API calls to be made in XML and be wrapped in a tightly structured envelope.
-
Remote procedure call (RPC) API: RPC API calls can be made in the HTTP and RPC programming languages, and function to trigger actions on remote servers.
Making API calls easier
It’s perfectly acceptable to manually code and input API calls but there’s no reason non-developers and less technical people can’t also make them. Here are few ways to make the API call process easier:
API best practices
Developers publish documentation to guide users of their API. The documentation often sets out a list of API best practices, including how to make successful API calls. You may choose to handle API calls through a centralized API management platform, for example, or use tools that are built-in to software to automate the call process.
Consult the relevant documentation in order to familiarize yourself with call methodologies for the API, and to troubleshoot any sticking points. Once you've read and understood the details, run test API calls to hone your application's interactions.
Wrapping up API calls
Now that you understand what API calls are, and how to use them, you’re ready to start making multiple requests using Contentful — and other types of APIs.
At Contentful, APIs don’t just power websites, but iOS and Android apps, and probably some of the smart devices you’re using around your home or office.
Up next: What is a headless API?
Written by
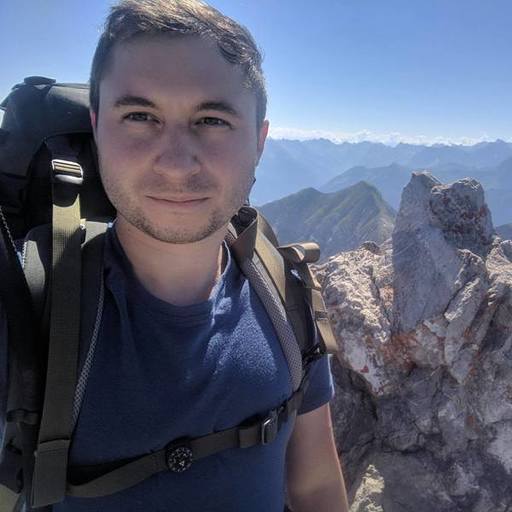
David Fateh
David Fateh is a software engineer with a penchant for web development. He helped build the Contentful App Framework and now works with developers that want to take advantage of it.