How to use Yup validation for HTML forms in React
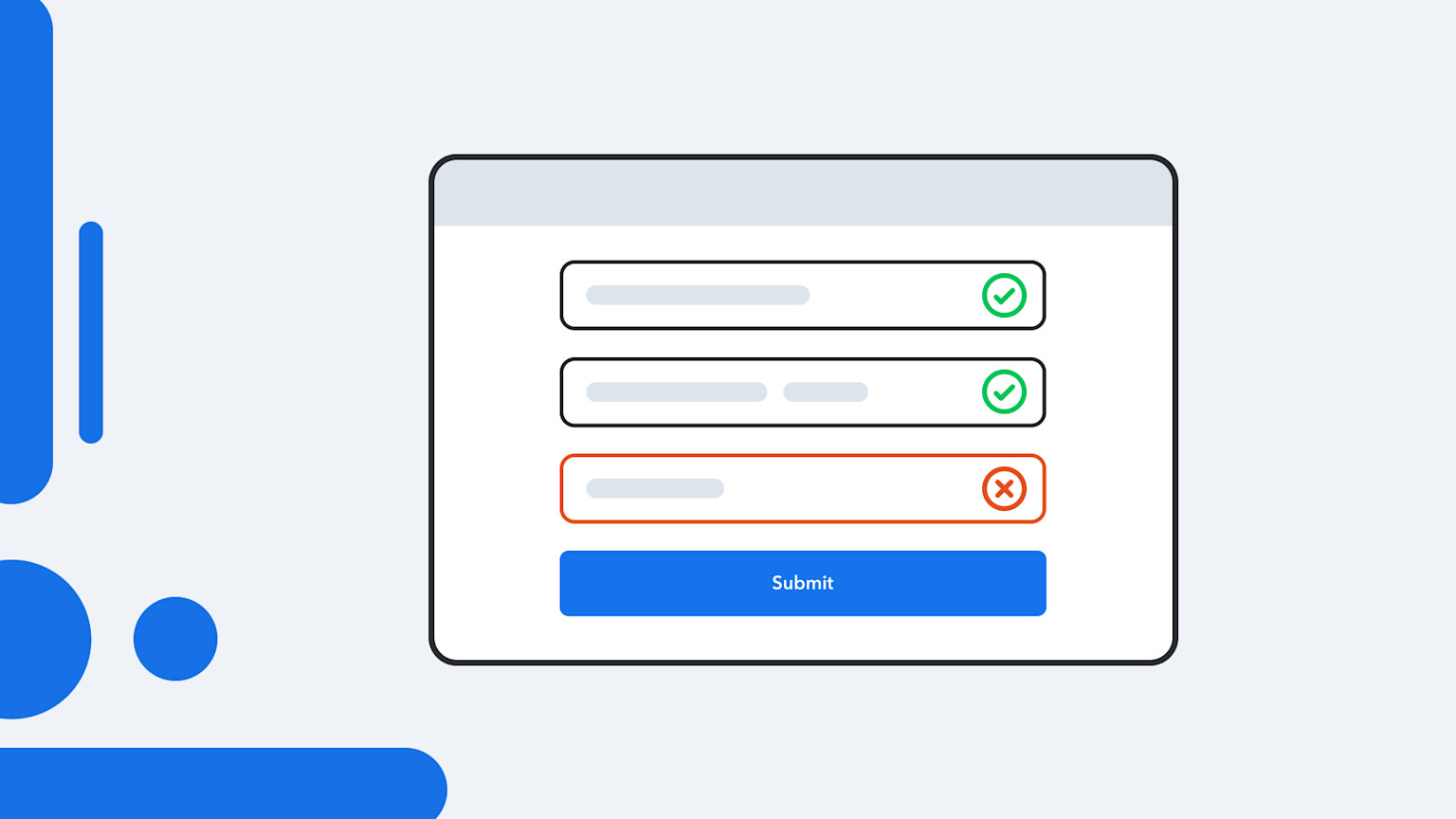
Client-side form validation provides your users with immediate feedback in case they incorrectly fill out a HTML form field. This enhances user experience by offering instant feedback, preventing application errors due to unexpected input values, and helps avoid unnecessary server requests that can slow down your app.
This tutorial shows you how to implement form validation in a React app using Yup, a JavaScript library you can use to validate data from user input.
What is Yup and why is it useful?
Yup is a JavaScript library that lets you build object schemas for your data, and then either validate your data to make sure that it matches the schemas or transform your data to conform to them. A Yup schema defines how the data should look and what kinds of values are expected.
Below is an example Yup schema that shows a user object consisting of three fields:
This schema defines that a user should have two string attributes (username
and password
) and a third boolean attribute indicating whether they've accepted the sign-up terms.
Yup has a number of built-in validation methods, which run tests over your data to check it conforms to the expected values. For example, you can check for a valid email address without having to write your own email validation code:
Yup also has parsing methods that transform your data into a preferred type or shape. This is often used to prepare data into a certain format before validating it. Below, a firstName
attribute is added that will have the leading and trailing whitespace removed using trim()
to ensure that a name is entered properly:
Yup makes your validation code easier to read and manage: defining all your validation rules in one place (a validation schema) makes it much easier to maintain your validation logic. It also makes it easy to chain validation rules together, allowing for code reuse:
Above, you can also see a custom error message has been added, which Yup allows you to define, so you can tailor them for your specific UX requirements. This could include providing error messages in different languages, or giving the user specific instructions based on why their input has failed validation based on other values in the form.
Yup can be used in any JavaScript application on the front or back end. One of its common uses is for validating HTML form data in apps developed with the React framework. For this, it integrates well with popular form libraries like Formik and React Hook Form.
How do you validate forms with Yup?
To validate forms with Yup you need to create a schema and then validate your data against it. Then, you can display any error messages to the user.
How to create a validation schema object
The schema object defines the shape that you want your data to conform to. For each field in your schema you can specify a number of validation tests, such as:
The data type: whether it’s a string, number, boolean, etc.
Whether the field is required.
If the field is a valid email address or URL.
You can also create your own custom tests to test anything you want.
Below is an example of a custom Yup test that checks if a string exactly matches an expected value.
Yup allows you to chain together as many validation methods as you need. Each schema method call returns a new schema object. Each schema object is immutable, so you can chain multiple schema methods together without them affecting each other.
You can also add Yup’s parsing methods to the chain of validation methods. For example, you can trim whitespace before validating a zip code:
How to validate data using a Yup schema
Once you’ve created a schema, you validate it by calling its validate()
method. This will then run all the tests defined within the schema.
If a test passes, it returns true
. If it fails, it returns a ValidationError
containing a message (this can be either a default or custom error message).
Using error objects to display Yup error messages to the user
Form validation error messages usually need to be displayed next to the relevant form input field. A simple way to deal with forms in React, including handling Yup validation and displaying errors, is to use Formik, a library for building forms in React that has its own ErrorMessage
component built in. With Formik, all you need to do is place the ErrorMessage
component below your form’s Field
component.
If Yup returns a validation error to Formik, it will be displayed after the input box. Let's look at a full example to see how all of this fits together in context.
Full code example: validating a HTML form in React using Yup
Below, we show how to configure Yup validation for a signup form on a React app. Options with a variety of different data types have been added to show the different types of validation that can be added.
Start with a basic create-react-app project. In your app.js
file, create a Yup schema for validating your form.
Next, you'll need to create the Formik form. Formik has its own <Formik>
component, which has a <Form
> component within it. You'll need to use these instead of React's standard <form>
component. Create a Formik form, and add the relevant Yup validation fields to this as you go.
The ErrorMessage component is responsible for displaying the validation error message. The name property should be the name of the field that the validation rule relates to.
If you want to add any special rendering to your error message (for example, have it displayed in red) you'll need to define this in your code by adding a class and then adding styling to that class:
If you want to follow along with our exact code, you can find this in our GitHub sign-up form with Yup validation GitHub repository. Be sure to check out the main src/app.js file for more form field validation examples such as dates, numbers, and a password confirmation field that needs to match the initial password.
To test this example, run the code in your browser by entering npm start into your terminal. In your browser, try entering different types of “bad” form data that would violate the Yup schema rules to see how the different error messages are displayed. For example, a username that's shorter than six characters could display the error message: "Must be minimum 6 characters".
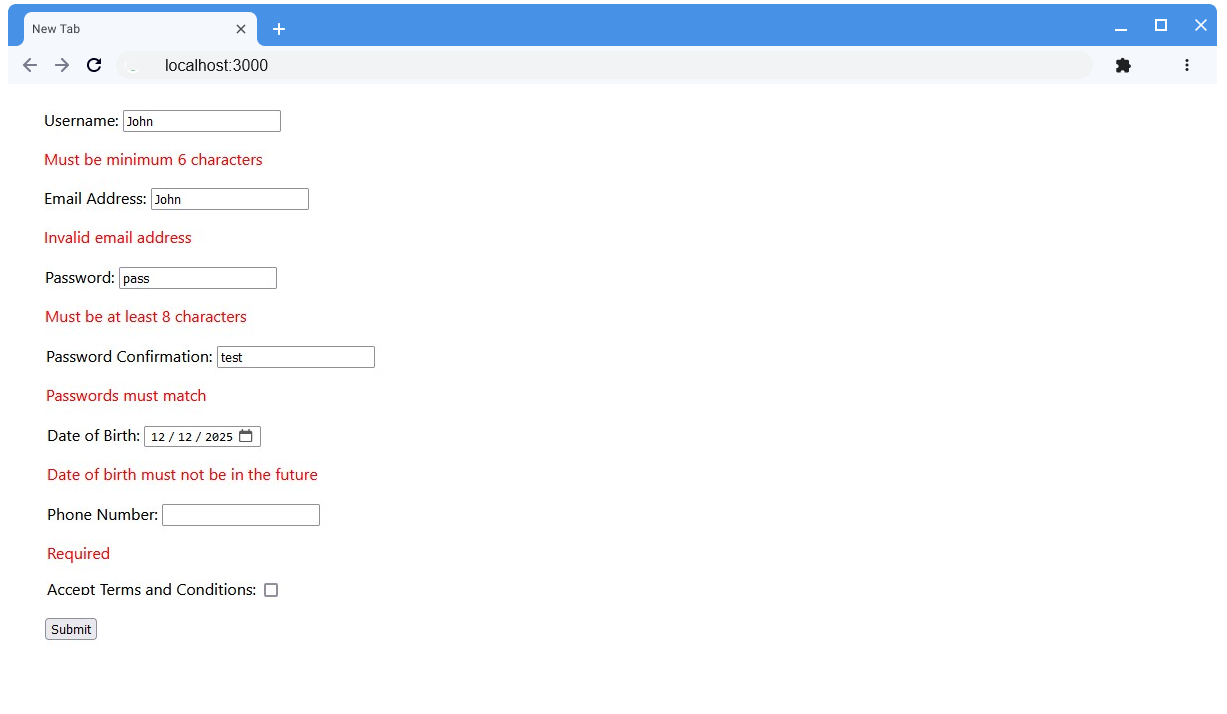
A variety of different error messages appear when the form input fields don't conform to the Yup schema validation rules defined above.
Do I need to use Yup with a library like Formik or React Hook Form?
Yup works as a standalone validation library. Strictly speaking, you don't need any extra libraries to make it work, as you can just use Yup with plain React. However, it's much easier to work with forms in React if you're using either Formik or React Hook Form, two popular libraries designed to make building forms simpler.
Yup can also be used for other types of validation, such as validating API request payloads, JSON being sent between backend services, or configuration data such as environment variables. It can even be used to transform data while it's being processed, to ensure it meets certain criteria before being used within an application.
In this tutorial, we focus on form validation — and we'll be using Formik throughout all the examples, as forms built using Formik support validation using Yup straight out of the box.
Form validation is important for a seamless user experience
The use of web forms can go beyond simply submitting data to be stored in a database. Forms are used in apps and websites to help users navigate and sort data, toggle options, and interact with content. For example, a recipe blog site might make use of a form to allow users to filter posts by category, date range, or words that appear in the recipe title:
In the Yup schema used to validate this search form, you might include a rule that ensures the titleContains
field contains between three and 20 characters:
… or that the category entered must be one of a predetermined list of categories:
… or that the published date must be between the date your blog started and the current date:
This is beneficial to both you and the user — you can ensure that valid filtering parameters are entered before making a request, preventing wasted bandwidth, and the user will be able to find what they are looking for faster, without seeing any errors from your back end if there's an unexpected value.
The benefit of using Contentful as a back end for your React apps
Like React with Yup validation, Contentful helps you build better apps faster by relieving you of a lot of legwork. It allows you to centralize your content production and publishing, so you only need to create your content once (like blog posts and ecommerce products) and use it across different channels, devices, and locales. You can use our GraphQL API to retrieve your content for your apps and websites, built with tools like React and Yup to provide fast, intuitive user experiences.
You can get started with Contentful by signing up for a free trial and following our helpful React starter tutorial.